Portals
Introduction
A portal is a single web application instance with preconfigured integrations with the ClearBlade system to which it belongs. It is fully responsive, mobile-friendly, and customizable.
Click here to learn how to get started with portals.
Purpose
Very powerful visual and interactive widgets to view and manipulate data
Role-based authorization
Out-of-box integrations with your ClearBlade system (e.g., fetching code services, maintaining tokens)
User-facing frontend for your ClearBlade system
Mobile-friendly
Real-time data streaming
User management
Highly customizable and extensible
Components
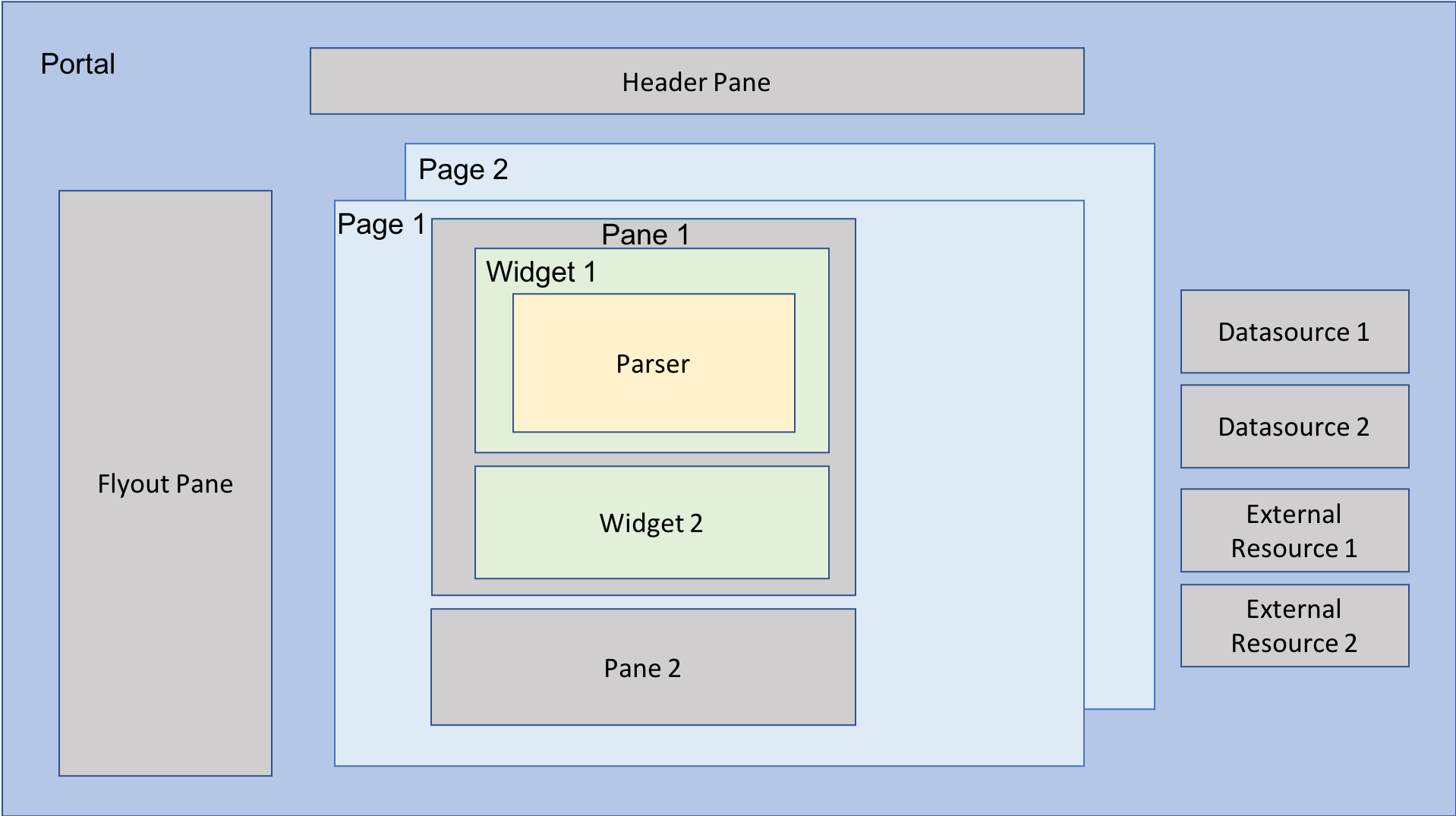
Version 7.0.0 and later:
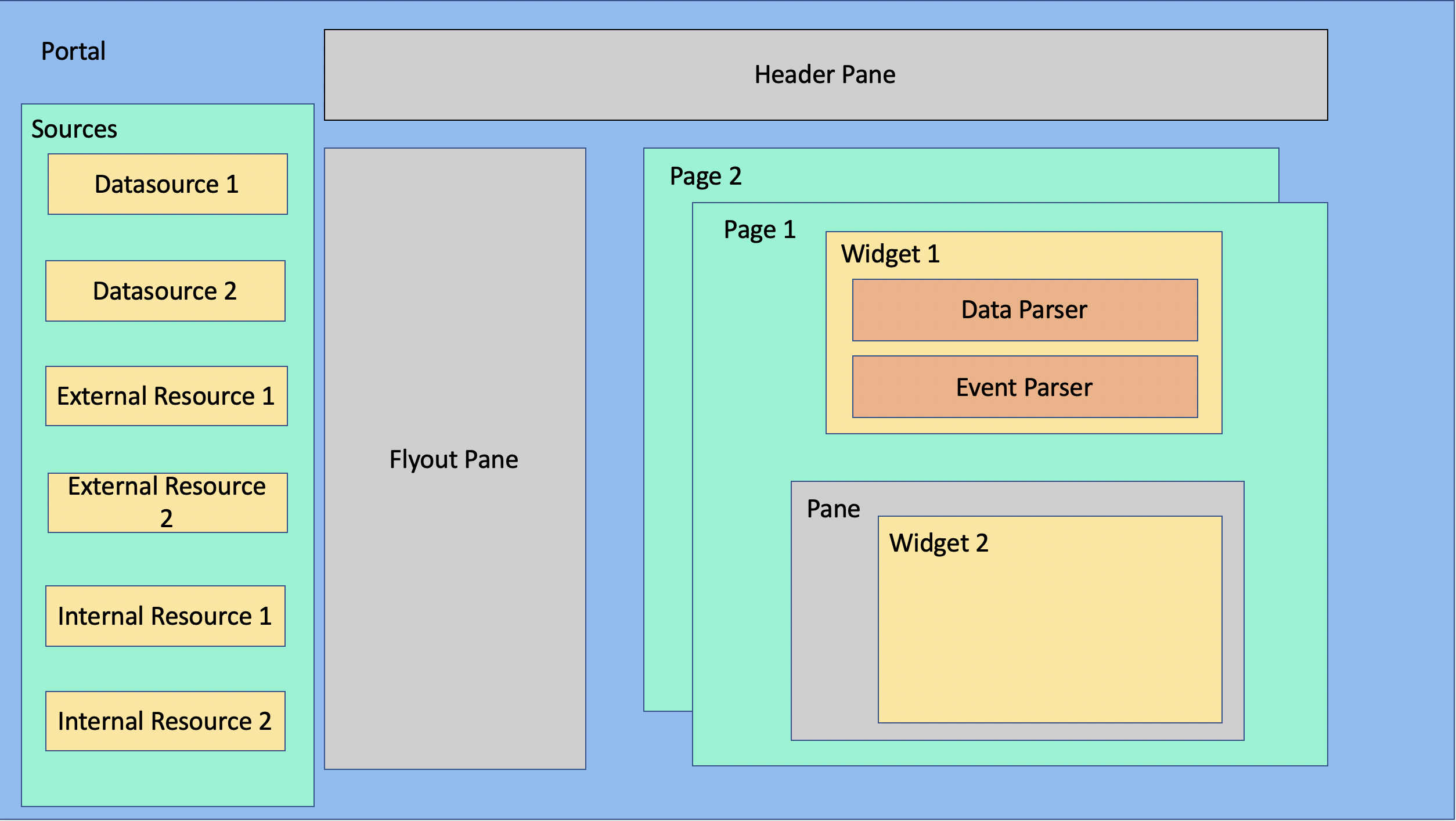
Datasource
Datasources are integrated with your system assets. They can consume or generate data, such as:
Datasources can be globally accessed by using the datasources
object.
Page
Users can view one page at a time. Every user starts on the home page by default. The page can change programmatically by running CB_PORTAL.selectPage from within a widget’s parser. A page can have multiple panes.
Pane
A pane is a container that holds one or more widgets. The pane’s width and height can be set according to Bootstrap grid.
Widget
A widget links to datasources and renders a visual or interactive way to manipulate them. Tutorials for each widget can be found here.
Parser
A parser manipulates a datasource's output before its ingestion by a widget. A datasource’s output may need to be modified into different data structures to be consumed by widgets.
Flyout pane
This customizable pane flies out from the left-hand side of the screen. The page can change programmatically by running CB_PORTAL.toggleFlyout from within a widget’s parser.
Header pane
The header pane is the same across all of a portal’s pages. It can contain multiple widgets.
Internal resource
An internal resource is a JavaScript or CSS file containing global functions or styles. This is loaded just once when the page loads. Add global/reusable code in this section, which the user chooses to be loaded before anything else loads on the page. Users can set the loading order of internal resources used on the portal. Users can also disable any resource. When this happens, they are not loaded. This is usually used while in development.
External resource
An external resource represents a web asset (such as JavaScript and CSS). Users can set the loading order of external resources used on the portal, and it can disable any resource. When this happens, they are not loaded. This is usually used while in development. This allows for a more customizable development of the widget using other resources.
Theme editor
You can choose or customize the portal’s color scheme.
Screen size editor
The pane and widget layout can be adjusted at different screen sizes.
Modal
This element sits on top of an application’s main window. It can contain widgets like a pane.
Security
The portal has a dedicated URL that can be shared and linked to a domain name. Users can visit a portal and interact with the data, edges, messaging, and other assets. A ClearBlade system user, such as an administrator, can be granted write access. This will allow them to customize a portal for another user.
Advanced
Plugins
A plugin is a JavaScript file that a portal can load to extend the default list of widgets and datasources. This can be used when a custom datasource is necessary and a custom widget is called for in a separate portal.
CB_PORTAL
This is used for portal management from the parser.
Local storage
Portals allow users to get/set values in the local storage using standard JavaScript functions. ClearBlade natively stores a few keys by default to avoid re-authentication and re-querying of constants.
Local storage stores:
Authentication information for the portal to avoid re-authentication
Platform and message URLs to avoid querying
Users can access it in a browser's local storage, usually via the Developer Tools' application section.
These are the pre-existing key-value pairs in local storage:
Key | Type | Description | Example |
---|---|---|---|
cb_messagePort | number | MQTT over the WebSocket port for messaging | 8904 |
cb_messageURL | string | Messaging broker | |
cb_platformURL | string | The platform that the broker is connecting to | |
cb_messageTls | boolean | The WebSocket port’s TLS status depends on the port being used. See here | true |
cb_portals | object | Users can set/unset their custom keys using the window.localStorage object in JavaScript |
|
cb_isEdge | boolean | Is the portal connected to the Edge Platform? | false |
Best practices
jQuery
Remove any element event handlers before adding a new one to avoid creating multiple handlers for one element.
FAQ
Settings
1. How do I change settings programmatically?
To get the settings programmatically, use
datasources.myDatasource.settings();
To set the settings programmatically, use
datasources.myDatasource.settings({
...datasources.myDatasource.settings(),
refresh_interval: 50})
This can be done in the widget’s parser.
Use the spread operator followed by properties/settings that must be changed programmatically.
Datasource
1. What are the different methods available on a parser’s datasource?
Users can access the selected datasource by using this.dsModel
, when using a dynamic
datasource. They can pair it with methods such as sendData
to call on them after being accessed.
E.g., this.dsModel.sendData(<DATA_TO_SEND>);
Datasources are used inside the widget parser, allowing users to use any of them.
Examples:
datasources.myDatasource.sendData(<DATA_TO_SEND>);
To retrieve the latest cache for a datasource, use
datasources.myDatasource.latestData()
To subscribe and unsubscribe to datasource updates, retrieve the data and assign it to a variable:
CODEconst updateCb = (data) { //do something with data } //then use: datasources.myDatasource.latestData.subscribe(updateCb) datasources.myDatasource.latestData.unsubscribe(updateCb)
if(datasources.myDatasource.lastUpdated){ const data = datasources.myDatasource.latestData(); }
To reach out to the server and update cache:
datasources.myDatasource.refresh()
2. How do I change a datasource’s message topic?
Create a new HTML widget and use your message topic as your datasource.
Edit the parser to include this code:
datasources.msg.settings({
...datasources.msg.settings(),
TOPIC_NAME: "newTopic"
})
Change msg and newTopic to your topic name and click save.
3. Can I set a datasource value to a false value to trigger any subscription callbacks for that datasource?
Yes, in ClearBlade Platform versions 7.0.6 and newer.
Widgets
1. How do I set up the rule builder’s permissions?
Permissions must be added to the system’s code services, triggers, and roles.

This can be done in the system’s roles section. Add a new role specifically for the rule builder.
2. How do I use the list widget to update other widgets?
Here is an example between the text and list widget:
Create a local variable
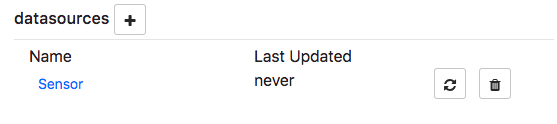
Add the list widget to the pane using the local variable as the selected item datasource.
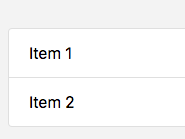
Add the text widget using the local variable as the datasource.
As you switch items on the list, the text will change to the item’s ID.
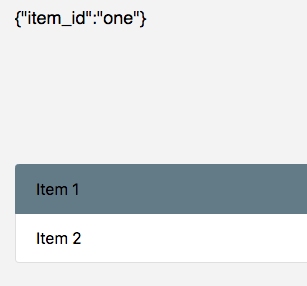
3. How do I render data from the HTML widget’s dynamic datasource?
Choose HTML from the widget type dropdown. Select Dynamic for the data type. Choose the datasource you want to use. Click Edit Parser. Add an id
attribute to the HTML parser, id="UniqueID"
. Type the following into the JavaScript parser:
parser = (ctx) => { document.getElementById('UniqueID').innerHTML=ctx.datasource; // If typeof ctx.datasource === "object" then user may have to select // the relevant key or stringify it. }
This is a collection datasource example. You can customize the widget to your preferences.