MQTT Broker Authentication
ClearBlade IoT Enterprise supports various types of authentication with the MQTT broker:
Auth Tokens
Obtaining a ClearBlade auth token over MQTT
A ClearBlade authentication token can either be obtained via a REST endpoint call (see: APIs ) or MQTT. To retrieve a token via MQTT, a separate connection must first be made on a different port, before continuing with your normal MQTT connection. See the table below for details.
Connect
Required keys | Description | Example values |
---|---|---|
URL | <PLATFORM_IP> | platform.clearblade.com |
Port | <PORT_NUMBER> | 8905 for non-TLS 8906 for TLS |
Username | <SYSTEM_KEY> | bacb8fb60bb4d7c2c2c0e4bb9701 |
*Password | <SYSTEM_SECRET> | BACB8FB60BFDDB7DB97D7A8BF01 |
ClientId | <USER_EMAIL>:<PASSWORD> for User <DEVICE_NAME>:<ACTIVE_KEY> for devices | cbman@clearblade.com:cl34r8l4d3 temperature-sensor:faqb8fb60bc2c2b1c0e4bb9701 |
Subscribe
To receive the new JWT token, subscribe to the auth topic (recommended).
To receive the legacy token, subscribe to the v/1/auth topic.
Extract token
ClearBlade publishes the user token on the auth message topic with bit-level encoding.
The payload is in this format:
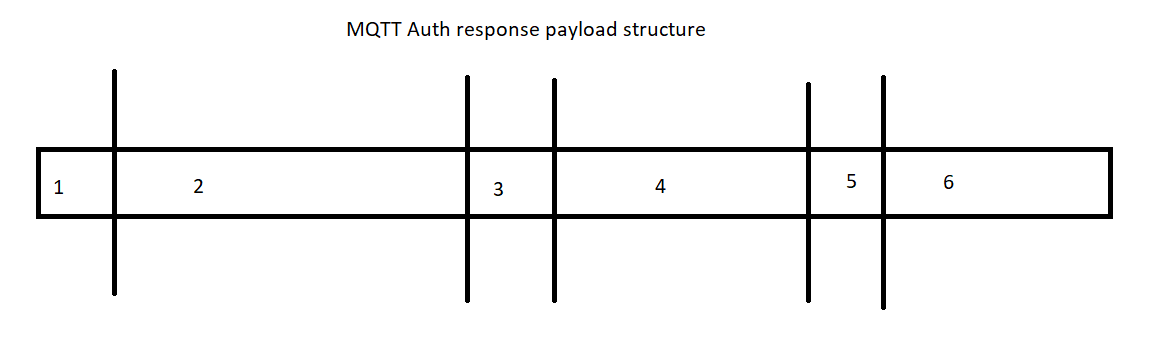
The length blocks are 2-byte unsigned 16-bit integers.
The data blocks are UTF-8 encoded strings.
Block mapping in the above packet structure:
Block-Num | Description |
---|---|
1 | Token length |
2 | Token |
3 | User ID or device name length |
4 | User ID or device name |
5 | Messaging URL length |
6 | Messaging URL |
Connecting to the broker with a ClearBlade auth token
Once the client is authenticated, the token can be extracted, and the connection can be established.
The MQTT protocol allows for the connect action to provide a username and password. We will modify the use of those fields to accommodate our OAuth-styled token model.
Key | Value | Example |
---|---|---|
URL | URL_OF_BROKER | platform.clearblade.com |
PORT | PORT | 1883 for non-TLS 1884 for TLS |
Username | USER_TOKEN | abcdefabcdef01234567890 |
Password | SYSTEM_KEY | f0cbf0cbf0cbf0cbf0cbf0cbf0cb |
ClientID | UNIQUE_CLIENT_ID | my-client-id-1 |
Asymmetric Keys
A device must create a private/public key pair. The private key is only left local on the device, while the public key is uploaded to the ClearBlade IoT Enterprise system’s device record.
See Creating key pairs to create the key pair.
To authenticate, the device will construct a JSON Web Token (JWT) based on the private key and present that on the MQTT authentication or REST endpoint’s connect packet. The JWT is used in place of the standard ClearBlade auth token.
The device uses the private key to sign the JWT. The token is passed to ClearBlade IoT Core as proof of the device's identity.
The service uses the device’s public key (uploaded before the JWT is sent) to verify the device's identity.
To add the public key to the device, use the following steps and example images:
Find the device in the system’s device table:
Right-click the device’s gear icon and choose Public Keys, then click the Add button:
Pick the appropriate key format:
ClearBlade IoT Core supports the RSA and elliptic curve algorithms. For details on key formats, see Public key format.
Claims
ClearBlade IoT Enterprise requires these reserved claim fields. They may appear in any order in the claim set.
Name | Description | Required for |
---|---|---|
| Issued at: The timestamp when the token was created, specified as seconds since 00:00:00 UTC, January 1, 1970. The server may report an error if this timestamp is too far in the past or future (allowing 10 minutes for skew). | MQTT, HTTP |
| Expiration: The timestamp when the token stops being valid, specified as seconds since 00:00:00 UTC, January 1, 1970. The token’s maximum lifetime is 24 hours + skew.
| MQTT, HTTP |
| System key: This must be a single string containing the ClearBlade registry’s system key. This can be obtained by clicking the API keys button at the top-right of the ClearBlade Registry Details page. | MQTT, HTTP |
| User ID: This must be a single string containing the deviceId. | MQTT, HTTP |
| User type: This must be an integer hard-coded to value 3. | MQTT, HTTP |
The optional nbf
(not before) claim will be ignored.
A JSON representation of the required reserved fields in a ClearBlade IoT Core JWT claim set is shown below:
{
"aud": "my-project",
"iat": 1509654401,
"exp": 1612893233
}
Only devices will be supported with this method.
Client type | Client ID | Username field | Password field |
---|---|---|---|
New ClearBlade client via JWT | Anything | -unused- | JWT token with sk, uid, and ut claims |
Each time the client sends an MQTT message (including PINGREQ), the ClearBlade MQTT Broker checks the exp. If the current time is later than exp + 10m then the client will disconnected. The 10 minutes is to allow for time skew between client and server.
The
generateJWT.py Python file shows an example of generating a JWT for the MQTT client’s password.
mTLS
Devices may connect using an mTLS approach to gain their access token. ClearBlade handles mTLS auth and TLS termination.
The mTLS configuration will be valid for all systems running in the IoT Enterprise instance.
Currently, no user interface administration is available to manage the loaded certificates. All certificates must be loaded to the server via API calls.
Requirements
The device name must be the cn (common name) in the cert presented. The platform verifies that the certificate passed in is for the device.
APIs
/admin/settings/mtls
GET, PUT (upsert), and DELETE support. Admin only.
PUT:
Body required:
{"root_ca": "certificate in PEM format. <required>", "crl": "certificate revocation list in PEM format. <optional>"}
Returns nil on success
GET:
No query support. Get the latest mTLS settings in JSON:
{"root_ca": "certificate in PEM format", "crl": "certificate revocation list in PEM format"}
DELETE:
No query support. Deletes mTLS settings.
/admin/revoked_certs
GET, POST, and DELETE support. Admin only.
POST:
Use to revoke a certificate. Body required:
{
“certificate_hash”: "sha256 hash of the ASN.1 DER format of the certificate <required>",
"description": <optional>
}
Returns nil on success.
GET:
Query supported. An empty query gets all revoked certificates, including those that match the query.
[
{"id": <id1>, "certificate_hash": <certificate1_hash>, "timestamp": <timestamp1>},
{"id": <id2>, "certificate_hash": <certificate2_hash>, "timestamp": <timestamp2>}
]
DELETE:
Query supported. An empty query deletes all revoked certificates and returns nil on success.
Authentication
A device can authenticate (i.e., receive a token) using mTLS by sending an HTTP POST request to port 444 of the relevant URL.
The following curl can be used as an example:curl -X POST "https://yourURL.com:444/api/v/4/devices/mtls/auth" -H 'Content-Type: application/json' -H 'Accept: application/json' -d '{"system_key": "yourSystemKey", "name": "yourDeviceName"}' --cert "path/to/yourDeviceCert.pem" --key "path/to/yourDeviceKey.pem"
The returned response will contain a device token.
Similarly, this Python code can be used as an example:
import requests
url = "https://yourURL.com:444/api/v/4/devices/mtls/auth"
headers = {"Content-Type": "application/json", "Accept": "application/json"}
data = '{"system_key": "yourSystemkey", "name": "yourDeviceName"}'
resp = requests.post(url, headers=headers, data=data, verify=True, cert=("path/to/yourDeviceCert.pem", "path/to/yourDeviceKey.pem"))
deviceToken = str(resp["deviceToken"])
ALPN (Application Layer Protocol Negotiation)
ClearBlade supports mTLS authentication for devices connecting via MQTT using ALPN on port 444. The ALPN protocol name is clearblade_mqtt_mtls
. Device Just-in-Time (JIT) provisioning is also supported. A Root CA certificate must be added via the mTLS admin endpoints before connecting devices via mTLS.
To connect to MQTT using mTLS, the device needs to set the following in the MQTT Connect packet:
The Username field must be a stringified JSON object with the device name set, amongst other column values, if required for JIT provisioning. For example:
'{"name": "myDevice"}'
The Password field must contain the system key.
Here’s a Python example using the Paho MQTT client:
from __future__ import print_function
import sys
import ssl
import time
import datetime
import logging, traceback
import paho.mqtt.client as mqtt
IoT_protocol_name = "clearblade_mqtt_mtls"
cb_iot_endpoint = "<CLEARBLADE_URL>" # For example test.clearblade.com
cert = "<DEVICE_CERTIFICATE_FILE>"
private = "<DEVICE_PRIVATE_KEY_FILE>"
username = '{"name": "device-1"}'
password = "<SYSTEM_KEY>"
logger = logging.getLogger()
logger.setLevel(logging.DEBUG)
handler = logging.StreamHandler(sys.stdout)
log_format = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s')
handler.setFormatter(log_format)
logger.addHandler(handler)
def ssl_alpn():
try:
#debug print opnessl version
logger.info("open ssl version:{}".format(ssl.OPENSSL_VERSION))
ssl_context = ssl.create_default_context()
ssl_context.set_alpn_protocols([IoT_protocol_name])
ssl_context.load_cert_chain(certfile=cert, keyfile=private)
return ssl_context
except Exception as e:
print("exception ssl_alpn()")
raise e
if __name__ == '__main__':
topic = "test/date"
try:
mqttc = mqtt.Client(client_id="<CLIENT_ID>")
ssl_context= ssl_alpn()
mqttc.tls_set_context(context=ssl_context)
mqttc.username_pw_set(username=username, password=password)
logger.info("start connect")
mqttc.connect(cb_iot_endpoint, port=444)
logger.info("connect success")
mqttc.loop_start()
while True:
now = datetime.datetime.now().strftime('%Y-%m-%dT%H:%M:%S')
logger.info("try to publish:{}".format(now))
mqttc.publish(topic, now)
time.sleep(1)
except Exception as e:
logger.error("exception main()")
logger.error("e obj:{}".format(vars(e)))
logger.error("message:{}".format(e.message))
traceback.print_exc(file=sys.stdout)
Shared Access Token Signature
Devices may authenticate using a shared access token signature.
Requirements
The shared access token should follow the signature outlined here. The token should not include a shared access policy and the resource URI should have the following form: <broker-domain>/devices/<system_key>/<device_name>
.
Devices using the azure SDK can be made to generate this format of token by setting the connection string as follows:
"HostName=yourdomain.clearblade.com;DeviceId=YourSystemKey/YourDeviceName;SharedAccessKey=YourKey"
The shared access key that the token is generated from must be uploaded to the platform using the following APIs.
APIs
/admin/devices/private_keys/<SYSTEM_KEY>/<DEVICE_NAME>
POST and DELETE support. Admin only.
POST:
Body required:
{"key_type": "shared_access_token", "private_key": "contents of base64 encoded shared access token"}
Returns the key on success
DELETE:
/admin/devices/private_keys/<SYSTEM_KEY>/<DEVICE_NAME>?id=key_id
Authentication
When sending a connect packet to the broker, the password must be the shared access token.
*System Secret was deprecated as of ClearBlade Platform version 9.38.0.